LoadChartView component displays allocations using a chart (and optionally an associated data grid) displaying normal and over-allocations of one or more resources through a project timeline. Supports custom appearance settings, built-in and custom scales.
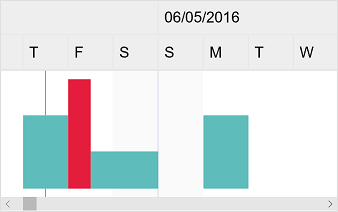
-
JavaScript®
-
TypeScript
-
AngularJS
-
Angular 8
-
React
-
Vue
Copy
Demovar loadChartView = document.getElementById("loadChartView"); var items = [{ content: "Resource 1", …, ganttChartItems: [{ content: "Task 1", start: new Date(…), finish: new Date(…), … }, { content: "Task 1, Task 2 [50%]", start: new Date(…), finish: new Date(…), units: 1.5, … }, …] }]; var settings = { itemHeight: 120, barHeight: 112, … }; DlhSoft.Controls.LoadChartView.initialize(loadChartView, items, settings); … loadChartView.refresh();
Copy
/// <reference path='./DlhSoft.ProjectData.GanttChart.HTML.Controls.d.ts' /> import LoadChartView = DlhSoft.Controls.ScheduleChartView; import ResourceItem = LoadChartView.Item; var loadChartViewElement = document.getElementById("loadChartView"); var items = <ResourceItem[]>[ { content: "Resource 1", …, ganttChartItems: [{ content: "Task 1", start: new Date(…), finish: new Date(…), … }, { content: "Task 1, Task 2 [50%]", start: new Date(…), finish: new Date(…), units: 1.5, … }, …] }]; var settings = <LoadChartView.Settings>{ itemHeight: 120, barHeight: 112, … }; var loadChartView = LoadChartView.initialize(loadChartViewElement, items, settings); … loadChartView.refresh();
Copy
<!-- AngularJS module dependency: DlhSoft.ProjectData.GanttChart.Directives --> <ds:load-chart items="items" settings="settings" auto-refresh="{{ true }}"> … </ds:load-chart>
htmlCopy
$scope.items = [{ content: "Resource 1", …, ganttChartItems: [{ content: "Task 1", start: new Date(…), finish: new Date(…), … }, { content: "Task 2", start: new Date(…), finish: new Date(…), units: 1.5, … }, …] }]; $scope.settings = { itemHeight: 120, barHeight: 112 … };
javascriptCopy
<!-- Dependencies: DlhSoft.ProjectData.GanttChart.HTML.Controls, DlhSoft.ProjectData.GanttChart.Angular.Components --> <loadchartview [items]="items" [settings]="settings"> … </loadchartview>
htmlCopy
import ResourceItem = LoadChartView.Item; var items = <ResourceItem[]>[ { content: "Resource 1", …, ganttChartItems: [{ content: "Task 1", start: new Date(…), finish: new Date(…), … }, { content: "Task 1, Task 2 [50%]", start: new Date(…), finish: new Date(…), units: 1.5, … }, …] }]; … this.items = items; this.settings = { … };
javascriptCopy
// Dependencies: DlhSoft.ProjectData.GanttChart.HTML.Controls, DlhSoft.ProjectData.GanttChart.React.Components items = [{ content: "Resource 1", …, ganttChartItems: [{ content: "Task 1", start: new Date(…), finish: new Date(…), … }, { content: "Task 2", start: new Date(…), finish: new Date(…), units: 1.5, … }, …] }]; … return <LoadChartView items={items} settings={…}>…</LoadChartView>;
javascriptCopy
<!-- Dependencies: DlhSoft.ProjectData.GanttChart.HTML.Controls, DlhSoft.ProjectData.GanttChart.Vue.Components --> <load-chart v-bind:items="items" v-bind:settings="settings"> … </load-chart>
htmlCopy
items = [{ content: "Resource 1", …, ganttChartItems: [{ content: "Task 1", start: new Date(…), finish: new Date(…), … }, { content: "Task 2", start: new Date(…), finish: new Date(…), units: 1.5, … }, …] }]; … var app = new Vue({ el: …, data: { items: items, settings: {…} } });
javascript
Items collection
To load and present data with LoadChartView control, initialize the items collection of the component, setting up objects representing resource rows identified by these main fields: content, and ganttChartItems. To present a single item, only add that one to items collection, and increase itemHeight and barHeight component setting values. Gantt Chart items collection defines timeline assignments and are identified by these fields: content, start, finish, and units.
Allocation items are presented as vertically positioned rectangular bars, having heights set to values proportional to assignment units. Over-allocations are highlighted by default, but you can also configure under-allocation visual hints.
Note that you can also prepare items for LoadChartView component from a GanttChartView instance using getLoadChartItems method.
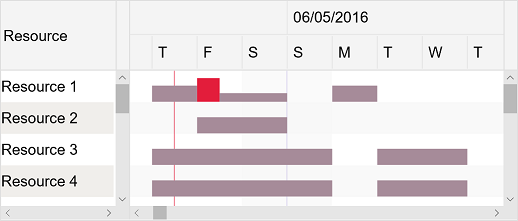
Copyvar items = [{ content: "Resource 1",
ganttChartItems: [{ content: "Task 1 (Resource 1)",
start: new Date(year, month, 2, 8, 0, 0), finish: new Date(year, month, 2, 16, 0, 0) },
{ content: "Task 1, Task 2 [50%] (Resource 1): 150%",
start: new Date(year, month, 3, 8, 0, 0), finish: new Date(year, month, 3, 12, 0, 0), units: 1.5 },
{ content: "Task 2 [50%] (Resource 1)",
start: new Date(year, month, 3, 12, 0, 0), finish: new Date(year, month, 4, 16, 0, 0), units: 0.5 },
{ content: "Task 3 (Resource 1)",
start: new Date(year, month, 6, 8, 0, 0), finish: new Date(year, month, 6, 16, 0, 0) }] },
{ content: "Resource 2",
ganttChartItems: [{ content: "Task 2 (Resource 2)",
start: new Date(year, month, 3, 8, 0, 0), finish: new Date(year, month, 4, 16, 0, 0) }] }];
DlhSoft.Controls.LoadChartView.initialize(loadChartView, items, settings);
Copyvar items = ganttChartView.getLoadChartItems();
DlhSoft.Controls.LoadChartView.initialize(loadChartView, items, settings);
Demo
Timeline
You may configure timeline duration, visible and working days and hours, scales, and zoom level (width of an hour) in the view. The component can further be zoomed in and out by moving the mouse wheel. Special nonworking days may be defined as a simple array of Date objects.
Copysettings.timelineStart = start; settings.timelineFinish = finish;
settings.visibleWeekStart = 1; settings.visibleWeekFinish = 5; // Mon-Fri
settings.workingWeekStart = 1; settings.workingWeekFinish = 4; // Mon-Thu
settings.workingDayStart = 10 * hourDuration; settings.workingDayFinish = 19 * hourDuration; // 10-19
settings.scales = [ { scaleType: "Months"; headerTextFormat: "Month", … } ];
settings.hourWidth = 5;
settings.specialNonworkingDays = [ new Date(…), … ];
Grid columns, rows, and changes
You may customize the column collection of the grid by resetting it or by adding and removing built-in columns and optionally defining new ones using JavaScript® functions initialized as column.cellTemplate. You may also filter items out by setting item.isHidden values to true and refreshing the user interface. To be notified when items managed by the component change either by editing grid cells or within the chart area, simply provide a handler function for settings.itemPropertyChanged.
Copysettings.isGridVisible = true;
settings.gridWidth = settings.chartWidth = "50%";
settings.columns = [
{ …,
cellTemplate: function (item) {
document = control.ownerDocument;
var element = document.createElement(…);
…
return element; } },
…];
settings.itemPropertyChangeHandler =
function (item, propertyName, isDirect, isFinal) { … };
Appearance
You may fully customize item appearance, either for the entire chart or for individual entries in the view, using CSS class mappings such as settings.normalAllocationBarClass, overAllocationBarClass, etc. (similar inline style settings are also available, if needed). Moreover, you may easily configure alternativeItemClass and alternativeChartItemClass settings for the grid and chart areas, and item.class and item.barClass fields also apply to the elements of any individual item that needs to be highlighted in the view.
Copysettings.overAllocationBarStyle = "stroke: Red; fill: Orange";
settings.alternativeItemStyle = "background-color: #f9f9f9"; settings.alternativeChartItemStyle: "fill: #f9f9f9";
items[i].ganttChartItems[j].barStyle = "stroke: Red; fill: #ffd54e";
Printing and exporting
The component offers a print method that may be called to initiate a direct print operation for their content. Moreover, you can export HTML out of a component content by calling exportContent methods on the fly. Both methods support custom configurations, such as indicating which grid columns, row range, and timeline period to be included as output. Also, setting up rotate field of the argument settings to true enables direct landscape printing.
CopyloadChartView.print({ title: "Load Chart (printable)",
isGridVisible: true, columnIndexes: [1],
timelineStart: new Date(…), timelineFinish: new Date(…),
preparingMessage: "…" });